Light¶
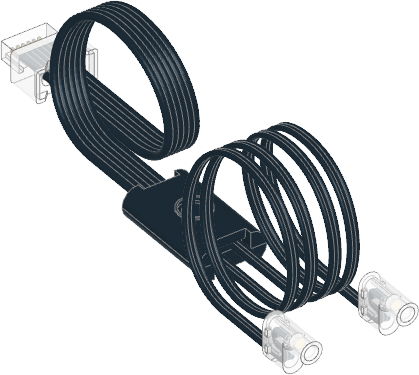
- class Light(port)¶
LEGO® Powered Up Light.
- Parameters:
port (Port) – Port to which the device is connected.
- on(brightness=100)¶
Turns on the light at the specified brightness.
- Parameters:
brightness (Number, %) – Brightness of the light.
- off()¶
Turns off the light.
Examples¶
Making the light blink¶
from pybricks.pupdevices import Light
from pybricks.parameters import Port
from pybricks.tools import wait
# Initialize the light.
light = Light(Port.A)
# Blink the light forever.
while True:
# Turn the light on at 100% brightness.
light.on(100)
wait(500)
# Turn the light off.
light.off()
wait(500)
Gradually change the brightness¶
from pybricks.pupdevices import Light
from pybricks.parameters import Port
from pybricks.tools import wait, StopWatch
from umath import pi, cos
# Initialize the light and a StopWatch.
light = Light(Port.A)
watch = StopWatch()
# Cosine pattern properties.
PERIOD = 2000
MAX = 100
# Make the brightness fade in and out.
while True:
# Get phase of the cosine.
phase = watch.time() / PERIOD * 2 * pi
# Evaluate the brightness.
brightness = (0.5 - 0.5 * cos(phase)) * MAX
# Set light brightness and wait a bit.
light.on(brightness)
wait(10)